아직까지는 iOS의 수많은 프로젝트가 UIKit기반일 듯 한데요.
그래서 Apple에서는 SwiftUI를 밀고 있으면서도
UIKit을 SwiftUI에서 재사용하거나,
최근(WWDC2022)에는 UIKit(UITableview)에 SwiftUI를 녹여서 쓸 수 있는 방법을 제시하고 있습니다.
그중에서 SwiftUI에서 UIKit을 사용할 수 있도록 제공하는 방법이
UIViewRepresentable, UIViewControllerRepresentable 프로토콜입니다.
UIViewRepresentable 프로토콜은 UIView를
UIViewControllerRepresentable 프로토콜은 UIViewController를 SwiftUI에서 사용할 수 있도록 해줍니다.
두 프로토콜들은 iOS 13.0부터 사용 가능합니다.
(각각의 Define을 스크린샷으로 담기엔 문서화 주석이 많아서)
프로토콜에서 꼭 구현해야 할 요소들만 정리를 해보자면 아래와 같습니다.
// UIViewControllerRepresentable 프로토콜
public protocol UIViewControllerRepresentable : View where Self.Body == Never {
associatedtype UIViewControllerType : UIViewController
func makeUIViewController(context: Self.Context) -> Self.UIViewControllerType
func updateUIViewController(_ uiViewController: Self.UIViewControllerType, context: Self.Context)
}
// UIViewRepresentable 프로토콜
public protocol UIViewRepresentable : View where Self.Body == Never {
associatedtype UIViewType : UIView
func makeUIView(context: Self.Context) -> Self.UIViewType
func updateUIView(_ uiView: Self.UIViewType, context: Self.Context)
}
사용 예시를 들어보겠습니다.
SomeViewController이라는 UIViewController를 작성했습니다.
SnapKit과 Then 라이브러리를 사용하여 작성했습니다.
(본 포스팅에 이어서 SnapKit 포스팅도 예정에 있습니다)
(Then 라이브러리 포스팅: https://swifty-cody.tistory.com/42)
class SomeViewController: UIViewController {
let someView = UIView().then {
$0.backgroundColor = .systemPink
$0.layer.cornerRadius = 10
$0.layer.borderWidth = 10
$0.layer.borderColor = UIColor.purple.cgColor
}
override func viewDidLoad() {
super.viewDidLoad()
self.drawSubviews()
}
func drawSubviews() {
self.view.addSubview(someView)
someView.snp.makeConstraints {
$0.width.height.equalTo(100)
$0.center.equalToSuperview()
}
}
}
그리고 SomeViewController를 위한 UIViewControllerRepresentable 프로토콜을 구현해보면 아래와 같습니다.
updateUIViewController(_:, context:)는 SomeViewController를 사용하는 SwiftUI에서 업데이트가 일어날 때 호출되는 함수로 SomeViewController가 변화에 대응할 수 있도록 해줍니다.
struct SomeViewControllerContainer: UIViewControllerRepresentable {
func makeUIViewController(context: Context) -> UIViewController {
let someViewController = SomeViewController()
return someViewController
}
func updateUIViewController(_ uiViewController: UIViewController, context: Context) {
}
typealias UIViewControllerType = UIViewController
}
이제 SwiftUI에서 SomeViewController를 사용할 준비가 끝났습니다.
SwiftUI를 빠르게 확인할 수 있는 방법은 바로 직전에 정리했던 포스팅에서 소개한 PreviewProvider입니다.
(PreviewProvider프로토콜 포스팅: https://swifty-cody.tistory.com/43)
PreviewProvider에서 SomeViewControllerContainer를 작성해보면 Preview에서 바로 확인해 볼 수가 있습니다.
struct SomeViewController_Previews: PreviewProvider {
static var previews: some View {
SomeViewControllerContainer().edgesIgnoringSafeArea(.all)
}
}
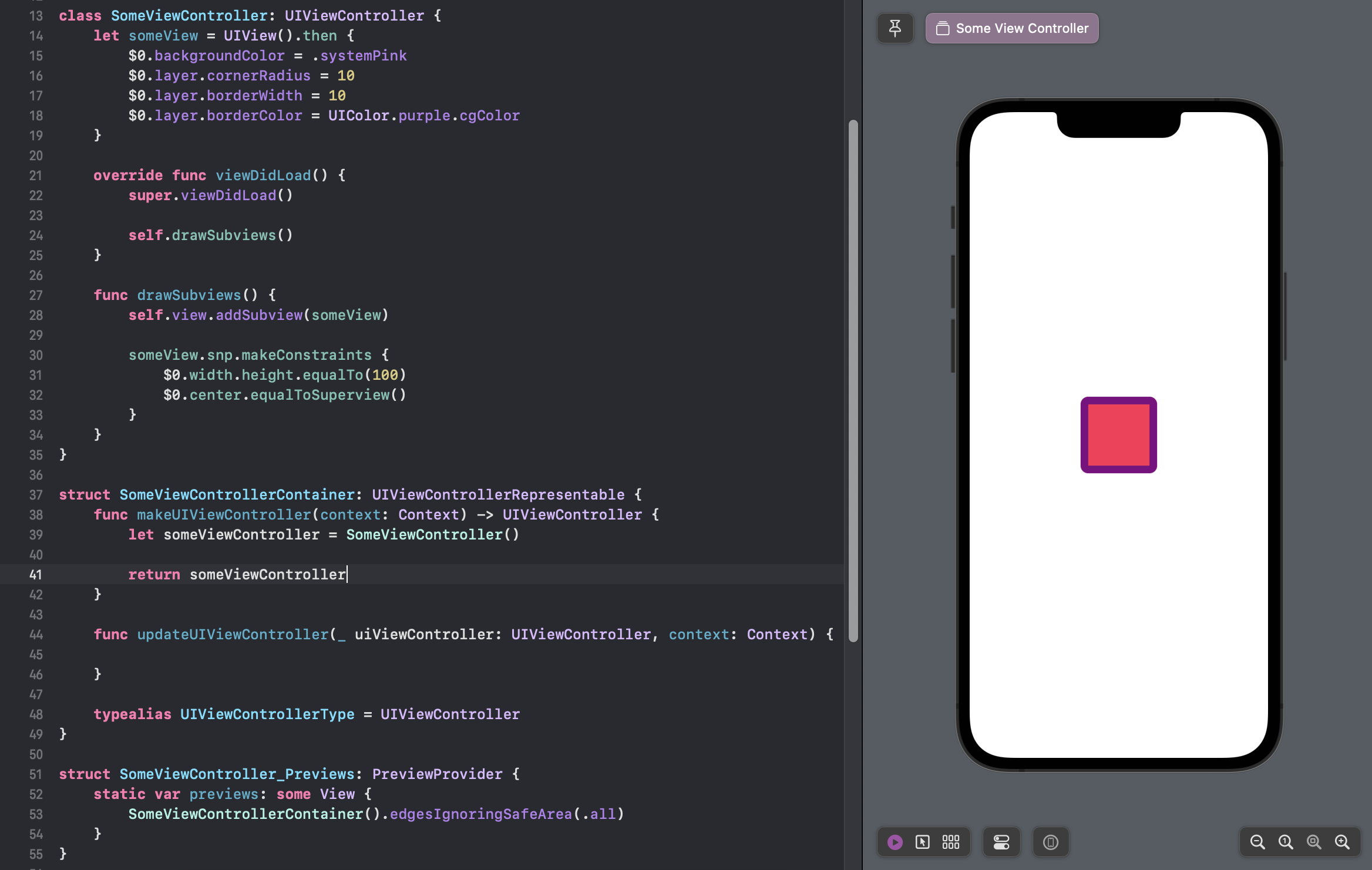
(UIViewRepresentable은 UIViewControllerRepresentable과 유사하기 때문에 생략합니다)
여기까지가 UIViewRepresentable, UIViewControllerRepresentable 프로토콜에 대한 포스팅이었습니다.
아직까지는 iOS의 수많은 프로젝트가 UIKit기반일 듯 한데요.
그래서 Apple에서는 SwiftUI를 밀고 있으면서도
UIKit을 SwiftUI에서 재사용하거나,
최근(WWDC2022)에는 UIKit(UITableview)에 SwiftUI를 녹여서 쓸 수 있는 방법을 제시하고 있습니다.
그중에서 SwiftUI에서 UIKit을 사용할 수 있도록 제공하는 방법이
UIViewRepresentable, UIViewControllerRepresentable 프로토콜입니다.
UIViewRepresentable 프로토콜은 UIView를
UIViewControllerRepresentable 프로토콜은 UIViewController를 SwiftUI에서 사용할 수 있도록 해줍니다.
두 프로토콜들은 iOS 13.0부터 사용 가능합니다.
(각각의 Define을 스크린샷으로 담기엔 문서화 주석이 많아서)
프로토콜에서 꼭 구현해야 할 요소들만 정리를 해보자면 아래와 같습니다.
// UIViewControllerRepresentable 프로토콜
public protocol UIViewControllerRepresentable : View where Self.Body == Never {
associatedtype UIViewControllerType : UIViewController
func makeUIViewController(context: Self.Context) -> Self.UIViewControllerType
func updateUIViewController(_ uiViewController: Self.UIViewControllerType, context: Self.Context)
}
// UIViewRepresentable 프로토콜
public protocol UIViewRepresentable : View where Self.Body == Never {
associatedtype UIViewType : UIView
func makeUIView(context: Self.Context) -> Self.UIViewType
func updateUIView(_ uiView: Self.UIViewType, context: Self.Context)
}
사용 예시를 들어보겠습니다.
SomeViewController이라는 UIViewController를 작성했습니다.
SnapKit과 Then 라이브러리를 사용하여 작성했습니다.
(본 포스팅에 이어서 SnapKit 포스팅도 예정에 있습니다)
(Then 라이브러리 포스팅: https://swifty-cody.tistory.com/42)
class SomeViewController: UIViewController {
let someView = UIView().then {
$0.backgroundColor = .systemPink
$0.layer.cornerRadius = 10
$0.layer.borderWidth = 10
$0.layer.borderColor = UIColor.purple.cgColor
}
override func viewDidLoad() {
super.viewDidLoad()
self.drawSubviews()
}
func drawSubviews() {
self.view.addSubview(someView)
someView.snp.makeConstraints {
$0.width.height.equalTo(100)
$0.center.equalToSuperview()
}
}
}
그리고 SomeViewController를 위한 UIViewControllerRepresentable 프로토콜을 구현해보면 아래와 같습니다.
updateUIViewController(_:, context:)는 SomeViewController를 사용하는 SwiftUI에서 업데이트가 일어날 때 호출되는 함수로 SomeViewController가 변화에 대응할 수 있도록 해줍니다.
struct SomeViewControllerContainer: UIViewControllerRepresentable {
func makeUIViewController(context: Context) -> UIViewController {
let someViewController = SomeViewController()
return someViewController
}
func updateUIViewController(_ uiViewController: UIViewController, context: Context) {
}
typealias UIViewControllerType = UIViewController
}
이제 SwiftUI에서 SomeViewController를 사용할 준비가 끝났습니다.
SwiftUI를 빠르게 확인할 수 있는 방법은 바로 직전에 정리했던 포스팅에서 소개한 PreviewProvider입니다.
(PreviewProvider프로토콜 포스팅: https://swifty-cody.tistory.com/43)
PreviewProvider에서 SomeViewControllerContainer를 작성해보면 Preview에서 바로 확인해 볼 수가 있습니다.
struct SomeViewController_Previews: PreviewProvider {
static var previews: some View {
SomeViewControllerContainer().edgesIgnoringSafeArea(.all)
}
}
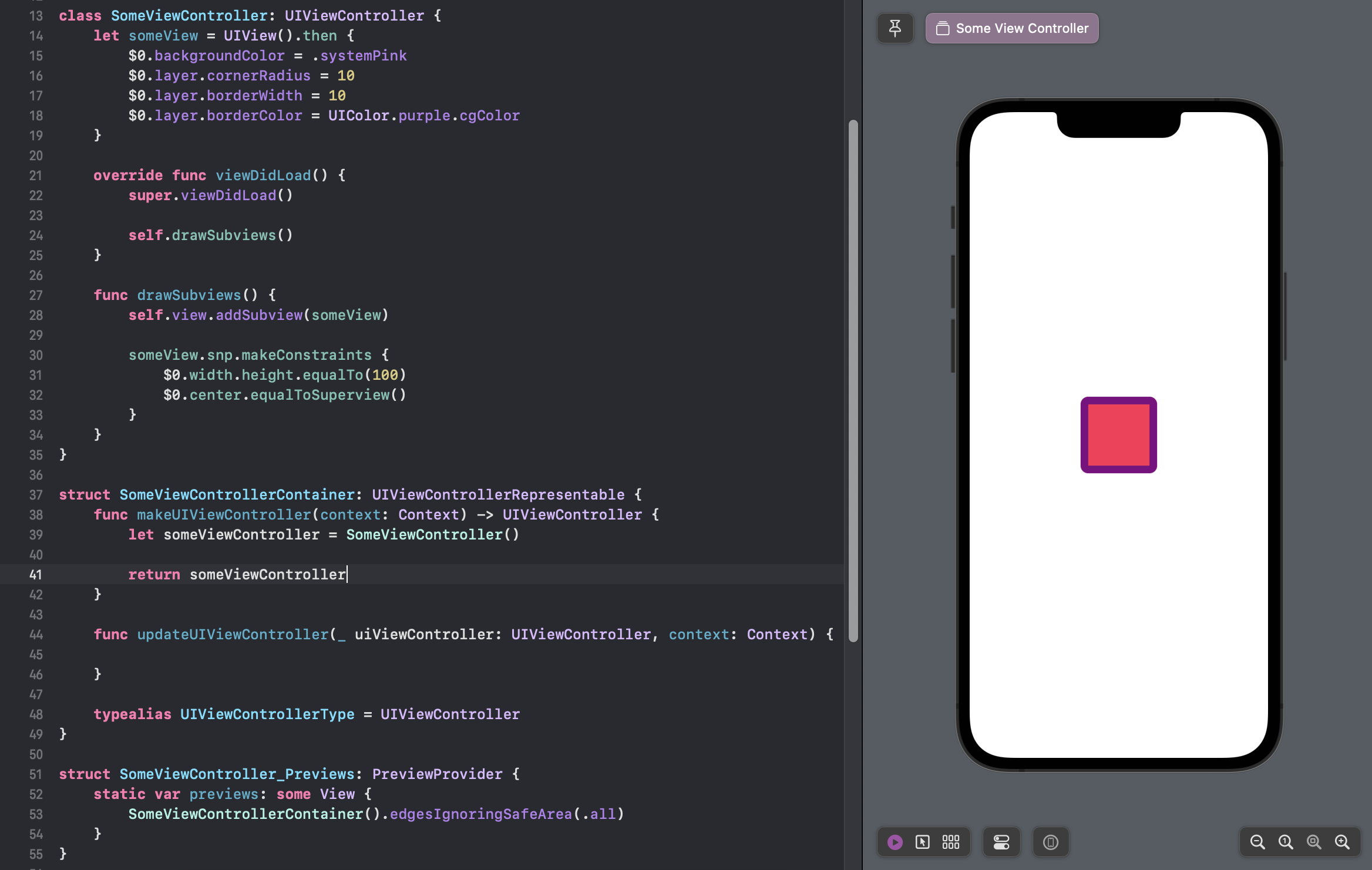
(UIViewRepresentable은 UIViewControllerRepresentable과 유사하기 때문에 생략합니다)
여기까지가 UIViewRepresentable, UIViewControllerRepresentable 프로토콜에 대한 포스팅이었습니다.